Sign your payments requests
Generate public and private keys and use them to sign API requests.
The Payments API v3 uses a pair of ECDSA keys in addition to a bearer token. The keys are a form of encryption that ensures your payments are secure.
With this form of encryption, you upload one public key to your Console account, and provide the other private key with your Payments v3 API requests. The combination of keys verifies and authenticates the request.
This procedure explains how to sign your requests.
1. Sign up for Console
Before you can sign your requests, you must sign up for a Console account.
2. Generate your key pair
To generate your key pair, you can use OpenSSL or LibreSSL, which are installed on MacOS and Windows by default. To do so:
- Open the command line.
- Use these commands to generate your key pair:
openssl ecparam -genkey -name secp521r1 -noout -out ec512-private-key.pem
openssl ec -in ec512-private-key.pem -pubout -out ec512-public-key.pem
The keys, named ec512-private-key.pem
and ec512-public-key.pem
by default, are generated in your current working directory.
Ensure that you store your private key securely.
To learn more about how to use the command line, see the Apple or Microsoft documentation.
3. Upload your public key to Console
- Go to Console > Payments > Settings.
- Upload your public key in the Signing keys box.
When you upload your public key, a KID is generated on this page. This is different to your public key itself, and is required to sign your requests.
4. Use a library to set up request signing
The end result of setting up request signing is that you can include a correctly formatted header named Tl-signature
with your requests. This header includes the private key you generated earlier.
In our Github, we maintain request signing libraries in a range of languages, so you can use the one best suited to your integration. Our other more general integration libraries also use these libraries for request signing.
For example, a Tl-Signature
looks like this in our Java request signing library:
// `Tl-Signature` value to send with the request.
String tlSignature = Signer.from(kid, privateKey)
.header("Idempotency-Key", idempotencyKey)
.method("post")
.path(path)
.body(body)
.sign();
Sign your requests without our libraries
It's possible to sign requests without using our libraries. The process is detailed here.
However, we strongly recommend that you use our signing libraries for easier integration.
Common request signing issues
When you set up request signing, make sure to check for these common issues.
- Whichever library you use, ensure that the
path
you sign is the same as the path used to send the request. For example, if you're testinghttps://api.truelayer-sandbox.com/test-signature
, the path must be/test-signature
. - All signed headers sent with a request must be exactly as they were signed, with none missing. So if you sign
Idempotency-Key
you must also send exactly the same value in the request. - Ensure that the body passed to the signing library matches the body sent with the request exactly, byte for byte. It must not be formatted differently or have fields in a different order. Ensure the request body has no trailing newlines if it was not signed that way.
- Ensure the public key and private key you are signing with match the KID (Public Key ID) in our Console. You can find your KID in Payments > Settings.
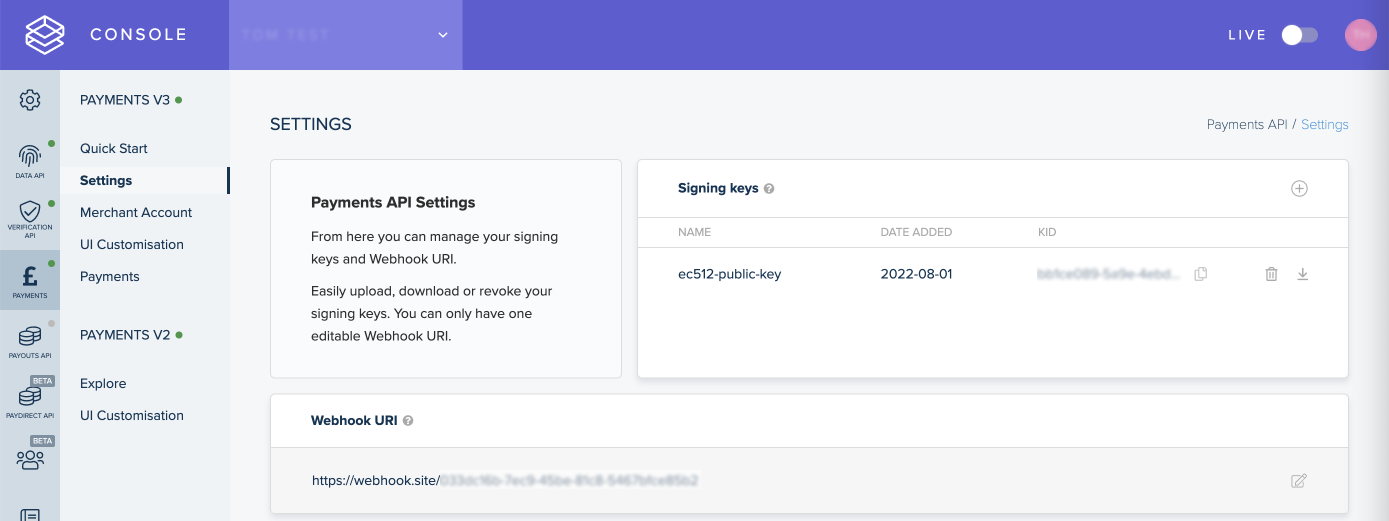
The Payments V3 > Settings page in Console, where you can upload your signing keys and add Webhook URIs.
5. Test your request signing
You can send a POST or DELETE request to our /test-signature
endpoint to check if your configuration works. If correctly configured, the endpoint validates your Tl-signature
and returns a 204 No Content
response. It doesn't validate your request body.
This is an example of a request to the /test-signature
endpoint:
curl -X POST \
-H "Authorization: Bearer ${access_token}" \
-H "Tl-Signature: ${signature}" \
--data '{"nonce":"9f952b2e-1675-4be8-bb39-6f4343803c2f"}' \
https://api.truelayer-sandbox.com/test-signature
Payments API signing libraries
We provide backend libraries and signing libraries in a variety of programming languages to simplify the integration process.
Request signing and validating webhook signatures are the most complex and time-consuming parts of integration for the Payments API. Client libraries help simplify this so you can get up and running faster.
Integration methods
Regardless of the integration method you choose, you need to handle some backend integrations. You can use client libraries with all of TrueLayer’s direct integration methods (web UIs, mobile app UIs, or a direct API integration).
Our libraries are available in two programming languages: Java and .NET. We regularly update them for breaking and non-breaking API changes.
To explore our client libraries and signing libraries, visit the TrueLayer Github.
Updated 10 days ago