Web SDK v0 to v1 migration guide
Upgrade from Web SDK v0 to v1.
Web SDK v1 is a new version of the Web SDK.
In Web SDK v1, the client first initializes the Web SDK (without a payment).
When the user taps the Pay button, we invoke the onStartFlow()
callback. When this happens, you can create the payment and pass it to the Web SDK.
This:
- reduces the number of payments that expire
- enables your user to go back and change their shopping cart amount after they have reached the checkout page
An example implementation of Web SDK v1 is below:
const { start, mount } = initWebSdk({
currency: 'GBP' | 'EUR',
clientId: 'your-client-id',
returnUri: 'https://example.org/return-page',
user: {
email: '[email protected]',
},
async onStartFlow() {
// `createPayment` is an example of your function (not part of the TrueLayer Web SDK)
// that makes a server call to create the payment
const { paymentId, resourceToken } = await createPayment()
// pass the `paymentId` and `resourceToken` to kick off the payment flow
start({ paymentId, resourceToken })
},
...
})
// mount the Pay button
mount(document.getElementById('your-container-id'))
New mandatory properties
When you make a payment with the Web SDK in any geography, you must pass:
- your client ID, in the
clientId
field - your end user’s email address, inside the
user
object.
You must also include a currency
field. This can be one of GBP
or EUR
.
You must include a return URI when you initiate a payment with the Web SDK.
To do this, supply a property inside the returnUri: 'https://example.org/return-page'
Provider filter object
You can use the providerFilters
object to control which logos appear on the Pay button at checkout. You can filter which logos appear by country and release channel, and can also supply provider IDs in the providerIds
field to show specific banks.
We recommend using this object if you are based in the EU.
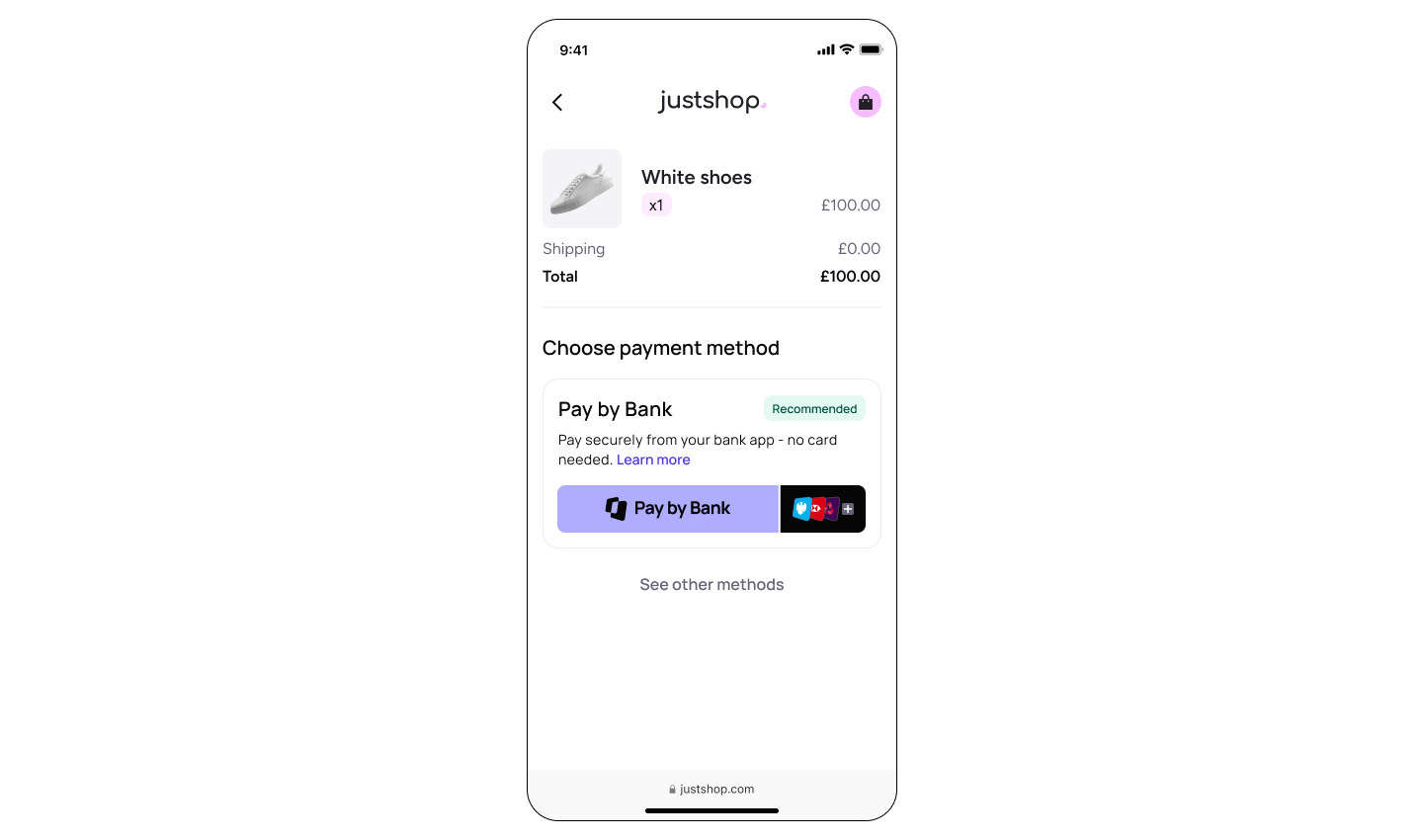
An example of a Web SDK checkout with a Pay button, including provider logos.
providerFilters: {
countries?: ['FR', 'DE', ...],
providerIds?: ['ob-monzo', 'xs2a-sparkasse', ...],
releaseChannel?: 'general_availability' | 'public_beta' | 'private_beta' | 'alpha'
}
If you’re in the UK, you don’t need to include this object (because we will only display UK providers).
Enable Signup+
Set the signupPlus
property to true
to render the Pay button and learn more page with Signup+ copy.
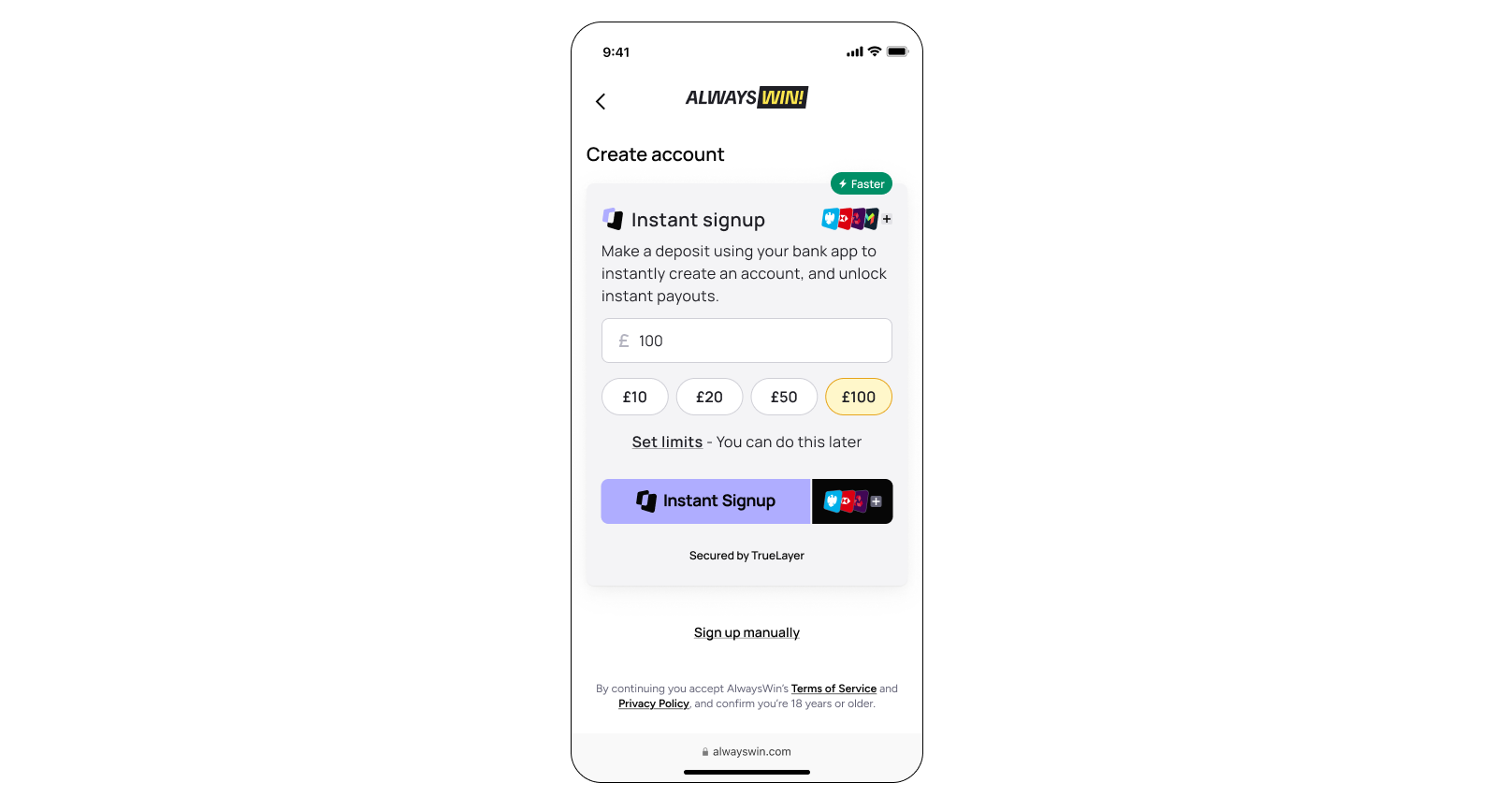
An example of a Web SDK checkout with Signup+ functionality.
Callbacks
Most of the callbacks are the same as Web SDK v0.
The exception is the onStartFlow() { ... }
callback. This is invoked when the user clicks the Pay button.
When it’s invoked, you need to:
- create a payment by calling your backend
- call
start({ paymentId, resourceToken })
to begin the payment authorisation flow.
Deprecated properties
hostedResultScreen: { returnUri }
This optional property is replaced by returnUri
.
onPayButtonClicked
This callback is replaced by onStartFlow
.
Updated 5 days ago