Web SDK
Enable users to authorise payments using the web SDK.
Web SDK v1
The Web SDK v1 is the newest version of the Web SDK. Upgrade for our most improved UX.
The web SDK is a quick way to integrate a TrueLayer authorisation flow into a webpage, which will enable you to embed a payments UI within your site. The web SDK provides our latest user experience optimised for conversion.
To integrate it, we recommend embedding our Pay button into your site. You must also implement payment retries.
The web SDK v0 is not compatible with VRP. If you have a third-party in-app web view (eg WebKit), the hosted payment page or mobile SDKs may be more suitable.
To see an example integration for the web SDK, jump to the Full request example section.
The user flow for payment authorisation
Below is an example of the steps that you and your user take to authorise a payment:
- The user selects the payment button.
- The web SDK uses this
id
andresourceToken
to begin the authorisation process. The authorisation screens displays as an overlay within your web page. - Your user selects their bank on the provider selection screen.
If a bank is unavailable, it's greyed out on the provider selection screen, so the user can attempt to use a different bank. - Your user enters any additional information that the bank requires, and confirms.
- If their bank supports app-to-app authentication, desktop users see a QR code. Scanning the code enables them to continue the payment using the banking app on their phone. They can also continue on desktop.
- The SDK redirects your user to their bank's website or app.
- Once the authorisation is complete, the bank redirects the user back to TrueLayer to show the payment result. The user will either:
- be taken to your
return_uri
if they were redirected to a new browser tab - close the web SDK modal if they remain in the original tab.
- be taken to your
- The user closes the modal and the webpage consumes the callback.
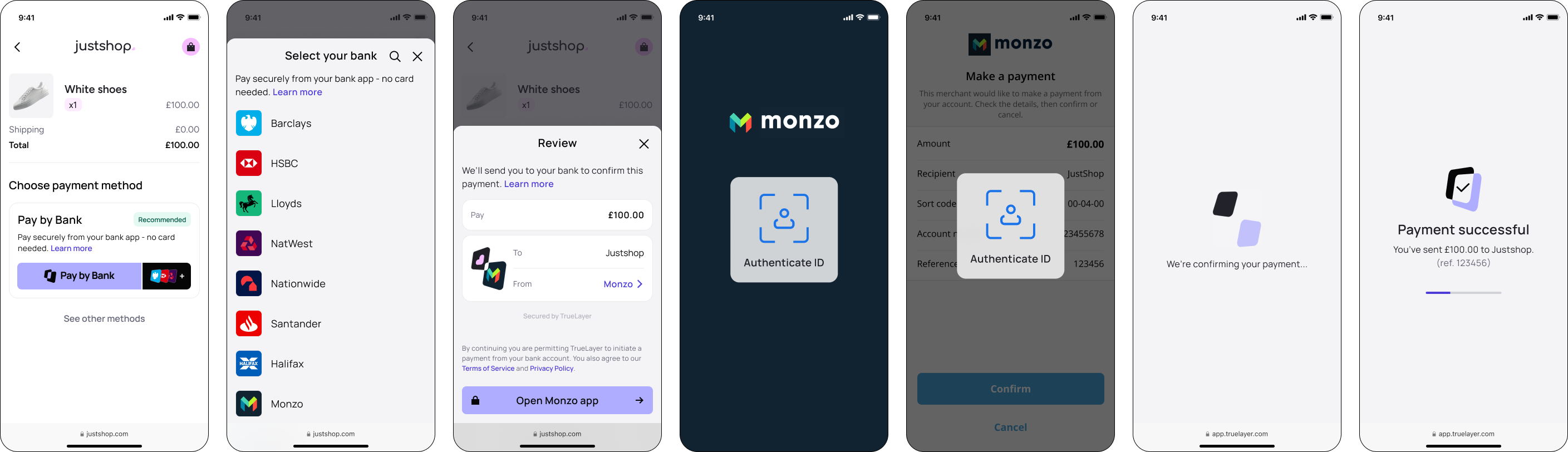
An example payment authorisation flow.
You can enable your users to save their payment details for a smoother journey when they pay you the next time.
Before you start
Firstly, you need to have an account in Console and be integrated with the Payments API v3.
Content security policy
If you have a content security policy, add TrueLayer’s domains to your allowlist to ensure that you can accept payments. These domains are:
- Sandbox:
https://app.truelayer-sandbox.com
- Live:
https://app.truelayer.com
Below is an example of a CSP HTTP header:
frame-src 'self' https://app.truelayer.com https://app.truelayer-sandbox.com
Dynamic Payment button
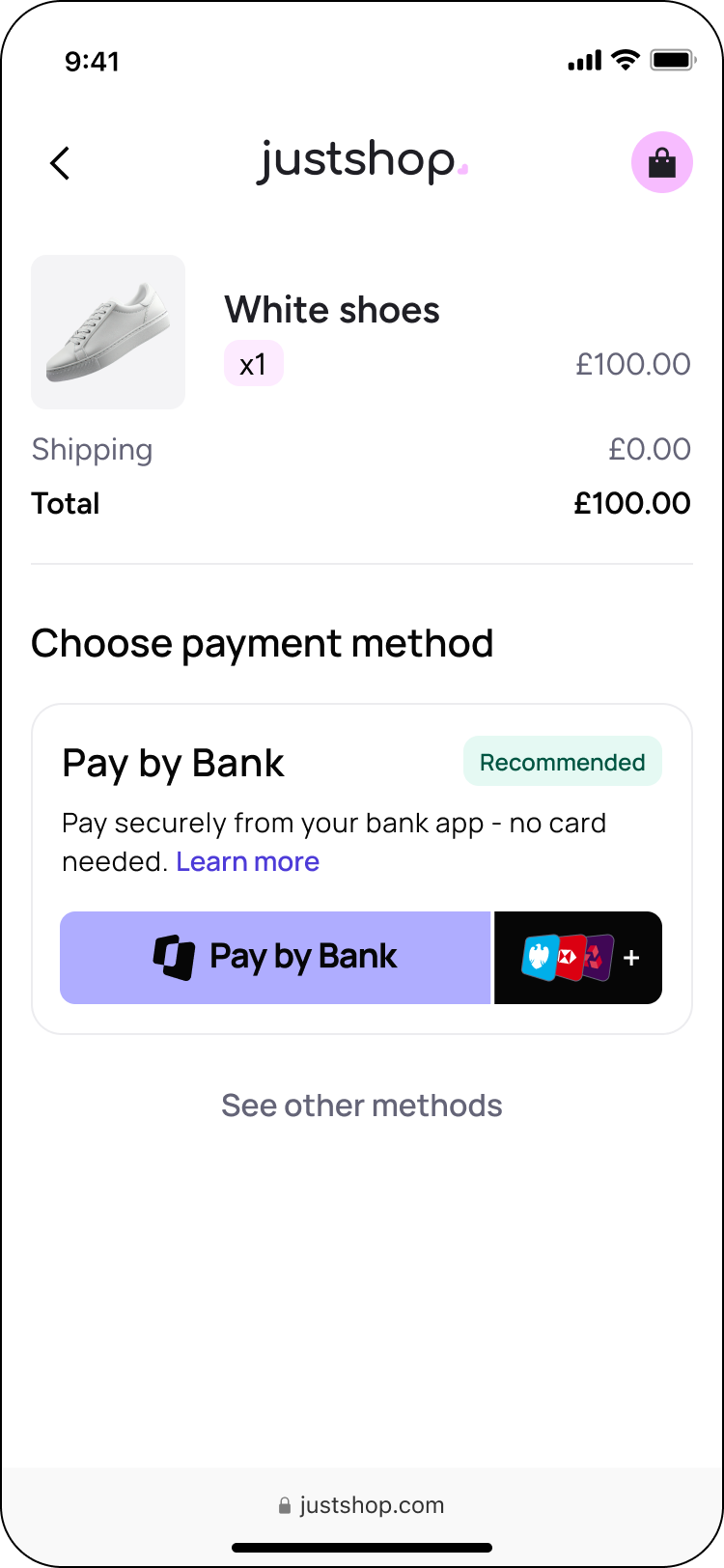
An example checkout screen with the web SDK enabled.
When your user makes a payment through the web SDK, you have the option of showing them a dynamic button at checkout, which contains the most relevant banks to them based on the banks they've used previously, or the most used banks in their market.
When the user clicks the button, they continue the flow in a modal on top of your page.
There are three size options to choose from when integrating the web SDK:
- The small option includes a Pay button only.
- The large option includes a Pay button and supporting information.
We recommend using this option, to help users understand the payment method. However, you also have the option to start the web SDK at a later stage, and use your own button at checkout. - The buttonless option enables you to configure your own button if your checkout does not support TrueLayer's dynamic button.
For more about changing the button size, or using the web SDK without the button, see Customise the Web SDK .
Payment retries for web SDK
To initiate a payment with the web SDK in the UK, we recommend that you enable payment retries. Retries enable several features that make the payment experience much smoother for your users.
If you are making payments in Europe, you do not need to set up payment retries. This will cause payments to fail.
To enable this feature, you need to include an empty retry
parameter in your payment creation request.
The retry object is located inside the payment_method
object within a Payments API request. It looks like this:
{
//...
"payment_method": {
"type": "bank_transfer",
//...
"provider_selection": {
//...
},
//...
"retry": {}
},
//...
}
Alternatively, contact us to get payment retries set up for your account.
When a retry fails, the payment moves into the attempt_failed
status. Like the authorizing
status, this payment status does not trigger a webhook, but you can make a GET request to monitor this payment.
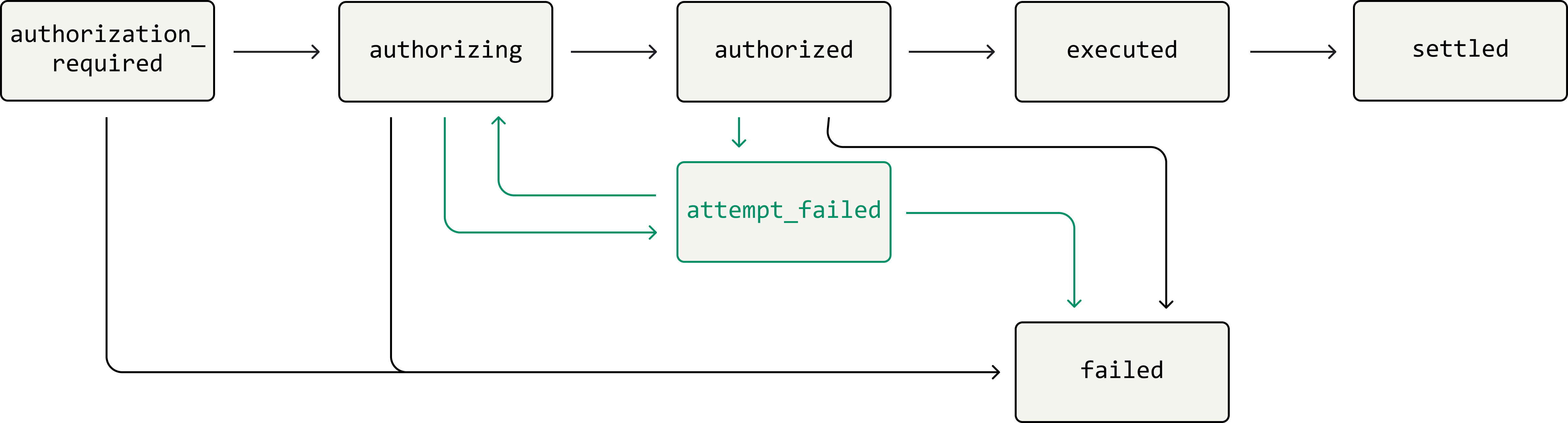
An illustration of the attempt_failed
status in the payment lifecycle.
Payments only move from attempt_failed
to authorizing
when a user retries a payment.
Your user can retry a payment as many times as they like before the payment expires in 15 minutes.
When retry isn’t available for a given payment:
- On the result screen for a failed payment, there are no Try again or Change bank buttons. Just close or done options remain. These no longer trigger a retry.
- On error screens, the Try again button is removed. This leaves only a close button, which no longer triggers a retry.
- On the QR code screen, the back button is removed.
- On the cancel form, there is no option for the user to change the bank that they want to pay with.
- If the user does choose to cancel the payment, then we call the
/cancel
endpoint. - If a user has paid before but chooses to add a new bank, there is only a close option and no back option on the list of their saved accounts.
- The payment button after the user cancels the payment is displayed in a disabled state.
None of the above changes impact the flow if the retry
object is present in the payment request.
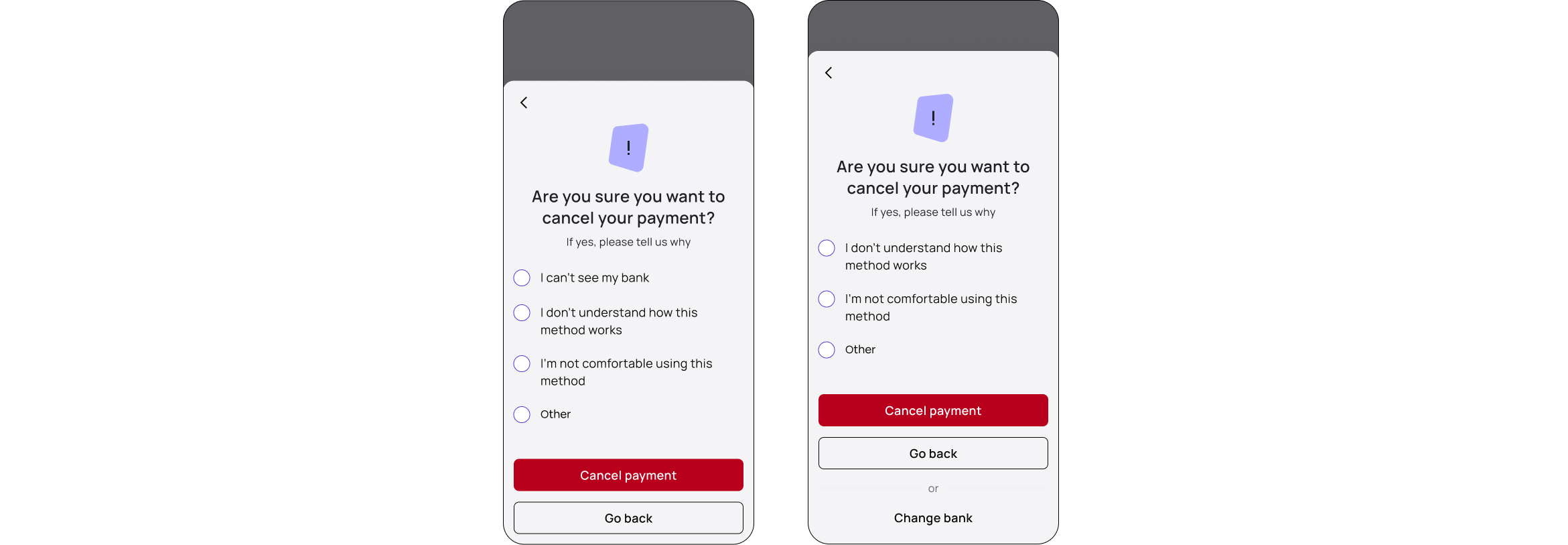
The difference between the cancel form without and with retries enabled.
The returning user flow
When your user has paid you for the first time, with a complete user
object, their details are saved. The appearance of the Pay button changes to show some of the saved account number for that user. If you are using your own payment button, the user still skips the provider selection screen. However, the checkout does not indicate the saved account details. Your user can save as many bank account details as they want, and choose which bank details to use for each payment. This functionality is enabled by default for new integrations.
This table describes each kind of user flow, which depends on whether your user is paying you for the first time or going through a returning journey.
User scenario | Payment flow | Pay button appearance |
---|---|---|
New user | User asked to select their bank on provider selection screen | SDK displays top bank logos, ordered by highest market share |
Returning user, saved account | Provider selection screen is skipped | SDK shows last used saved account, with the option to switch to other saved accounts from a dropdown menu |
Change provider without restarting the flow
If you have enabled retries, your user can click the Change bank button on the cancel form to go back to provider selection and pay with a different bank instead.
This feature is enabled by default for any Web SDK integration with retries enabled. If you do not have retries enabled, the button will not appear. Your user will have to end and restart the payment flow if they want to use a different bank.
How to integrate the web SDK
The web SDK is a JavaScript library, which means you can integrate in two ways. You can use a package manager, like NPM, or inject it to a HTML webpage using script tags.
Integrate the web SDK with a package manager
Use these commands to set up the Web SDK with the NPM, Yarn, or PNPM package managers:
- NPM:
npm i truelayer-web-sdk
- YARN:
yarn add truelayer-web-sdk
- PNPM:
pnpm install truelayer-web-sdk
Integrate the web SDK with Script tags
If you want to integrate the web SDK using script tags, it is hosted on the jsdelivr and unpkg CDNs.
You can access the latest version of the web SDK at these URLs:
- jsdeliver:
https://cdn.jsdelivr.net/npm/truelayer-web-sdk/dist/sdk.min.js
- unpkg:
https://unpkg.com/truelayer-web-sdk/dist/sdk.min.js
If you need a specific version, use these links and specify the version in place of the text {version}
:
- jsdelivr:
https://cdn.jsdelivr.net/npm/truelayer-web-sdk@{version}/dist/sdk.min.js
- unpkg:
https://unpkg.com/truelayer-web-sdk@{version}/dist/sdk.min.js
Create a payment and initialise the SDK
You need to pass a payment ID and resource token to initialise the web SDK. To do this, you need to create a payment.
We recommend that you do this when you load the checkout page. This leaves the shortest time possible between payment creation and initialising the SDK, which avoids issues related to payment expiry.
At the highest level, to accept a payment with the web SDK, you need to:
- create a payment
- initialise the SDK
- mount the container where the Pay button will be
- initiate a payment, obtain its
id
andresource_token
and pass these to thestart
function with yourreturn_uri
- have your user authorise the payment
- run the
cleanup
function after the payment is complete to remove all event listeners before re-initialising the SDK - handle any payments which expire
To initialise the SDK, you need:
- the payment
id
- the
resource_token
- the
return_uri
These are each handled by different functions. mount
displays the button in a loading state. The start
function activates the button and displays the correct bank logos.
Below are two examples:
initWebSdk({
hostedResultScreen: {
returnUri: merchant_return_uri,
},
//...
})
.mount(node)
.start({
paymentId: payment.id,
resourceToken: payment.resource_token
})
const { mount, start, cleanup } = initWebSdk({
hostedResultScreen: {
returnUri: merchant_return_uri,
},
})
// later, when the target for the button is ready
mount(node)
// later, when the payment is available
start({
paymentId: payment.id,
resourceToken: payment.resource_token,
})
For optimal user experience, we recommend that you mount the container and initiate the payment as early as possible when the user arrives at your checkout page.
Supply a returnUri
returnUri
Specify a URI to redirect users to after they complete the web SDK flow.
To do this, include the hostedResultScreen
object and supply a returnUri
property inside it.
To do this, include the hostedResultScreen
object and supply a returnUri
property inside it.
{
hostedResultScreen: {
returnUri: example_return_uri,
},
}
If you supply a hostedResultScreen
object with no return URI inside, the payment fails.
The web SDK is a UI that is embedded into your site or app, but users see the redirect animation and result screen on a hosted page. After that, they are automatically redirected to the return URI that you chose without interrupting the flow.
Note that your user will have two tabs open in their browser at the end of this process. One is the page where the user completed the payment. one is the checkout entry point. If they go back to the checkout, they will still see the Web SDK status page. The result screen is displayed as a full page, and not a modal.
If the payment fails and your user chooses to retry the payment, they restart the flow on the hosted payment page, instead of the Web SDK.
Handle payment expiry
Because you create a payment before you initialise the SDK, a payment might expire before the user can complete the flow. For example, if the user pauses during the authorisation flow to do something else, the payment may expire by the time they return to the flow. Payments expire after 15 minutes if the user doesn't start the authorisation flow.
In the UK, the retry feature allows users to retry failed payments within that 15-minute window, but beyond that a new payment will need to be created for the user to continue.
We recommend that you track this timeout period, and if necessary re-initialise the web SDK with a new payment once it expires. You can track the expiry either with your own timer, by using the exp
property of the resource token, or by using the onExpired
callback. This property has a timestamp that marks when it expires.
Re-initialise the Web SDK
To re-initialise the Web SDK, call the initWebSdk
function again with a new payment id
.
If the Web SDK encounters an error and needs to restart, which you can determine from the onError
callback, you also need to re-initialise the SDK.
Below is an example:
cleanup()
initWebSdk({ ... })
mount(node)
start({
newPaymentId: payment.id,
resourceToken: payment.resource_token,
})
Determine the outcome of a payment
Once the payment flow is complete, the user returns to your environment either by closing the web SDK modal or by being redirected to your return URI. If the user arrives from the SDK modal, then you receive an onDone
callback, which describes the result shown to the user.
From this moment, we advise you to check for payment status webhooks from the Payments API to determine the outcome of the payment.
A payment is safe to act on when it settles in your account. If you use a TrueLayer merchant account, you'll receive a payment_settled
webhook to indicate this.
However, in some cases you need to wait for a payment to settle. However, in some cases you need to wait for a payment to settle. For example, if you want to treat a payment as complete and actionable, configure the payment_creditable webhook.
Callbacks
You can include a set of callbacks within the initWebSdk
function to inform you when certain events in the authorisation flow have been completed (or when the user chooses to cancel the payment).
These are:
-
onPaymentButtonClicked
: The user selected the TrueLayer Pay by bank app payment method. -
onStartFlow()
(v1 only): The user has begun the payment authorisation flow. At this point, you can initiate a payment. You receive this callback only if you've updated to Web SDK v1. To enable the newest version, see the migration guide. -
onDone
: The Web SDK flow is complete. This can return three different results:String received Payment status or webhook it corresponds to 'success'
The payment meets the definition of creditable.
If you haven't configured thepayment_creditable
webhook, then by default a payment is treated as creditable once it reaches thesettled
status.'pending'
We're waiting for the bank to finalise the payment. It hasn't yet met the definition of creditable. Usually corresponds to the authorized
orexecuted
statuses .'failed'
The failed
status . -
onCancel
: The user exited the authorisation flow. This does not mean the payment itself is cancelled. The user can restart the flow by click on the payment button again. -
onError
: An unexpected error occurred and it isn't possible to process the payment. -
onExpired
: A callback function the SDK returns when the paymentresource_token
expires. You won't receive this if the payment status transitioned to a successful state. -
onNavigation
: A callback function the SDK returns when the user visits a new page. It returns a string that represents the page name, which can have one of the following values:String received Position in authorisation flow it corresponds to 'checkout'
Your checkout page. 'learn-more'
The "Learn more" modal. 'cancel'
The user exits the payment flow. 'providers'
The provider selection screen. 'account-selection'
The bank account selection screen. 'consent'
The consent screen. 'waiting-bank'
Waiting for their banking provider to authenticate. 'qr-code'
The QR code handoff screen. 'qr-code-loader'
The loader screen on desktop after the user has handed off to pay on mobile. 'return-from-bank'
Redirect back to your app after the user has authorised or cancelled the payment, or if authorisation fails.
Location of the onNavigation
callbacks in the flow
onNavigation
callbacks in the flowThese are examples of the screens that each of the onNavigation
callbacks respond to within the TrueLayer payment authorisation flow:
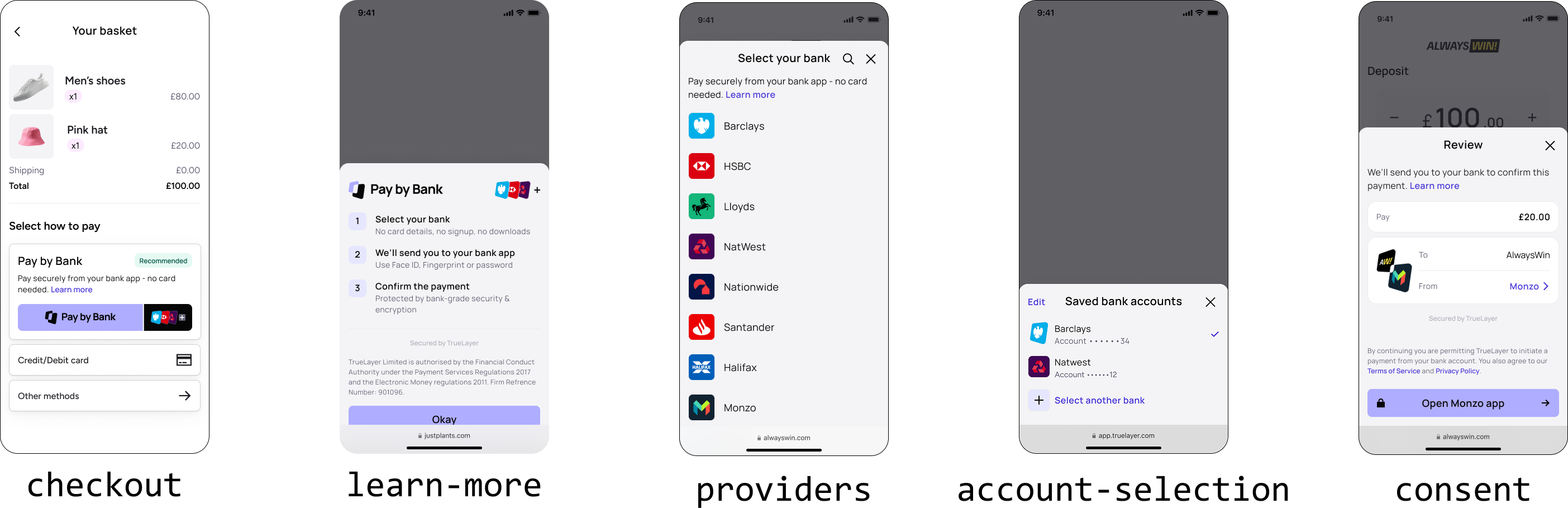
The screens in the mobile authorisation flow that each callback corresponds to.
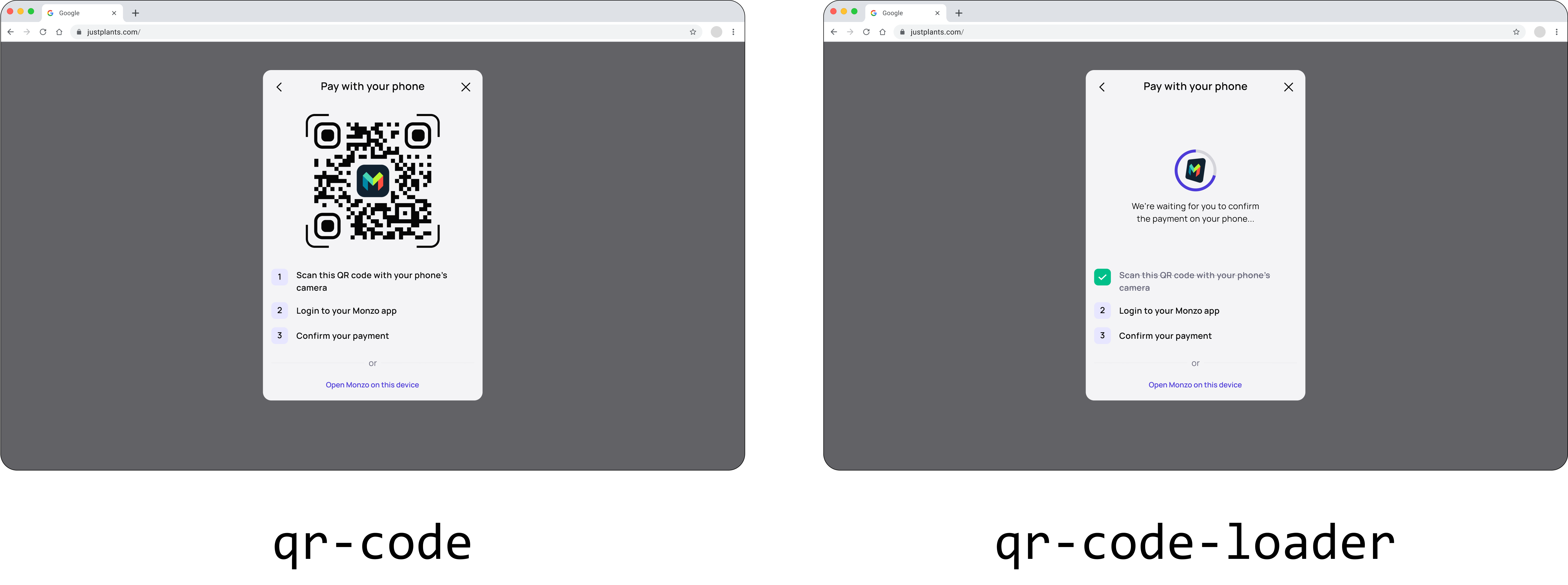
The QR code and handoff screens in the authorisation flow, alongside the callbacks which correspond to them. Note that the QR code handoff only applies to flows on desktop, not mobile.
Wait to complete a payment
You may want to allow more time for the payment to transition to a terminal status (settled
or failed
) before we redirect the user back to you. This is useful if you want to maximise the number of payments that have completed by the time the user returns to your screen.
To do this, include a maxWaitForResult
field within the initWebSdk
function and add a number to represent the maximum waiting time in seconds. This value controls how long the user waits before they are shown a result screen, if the payment has not reached a terminal status.
If you specify a higher maxWaitForResult
, it's likely you'll receive a result of settled
for the onDone
callback.
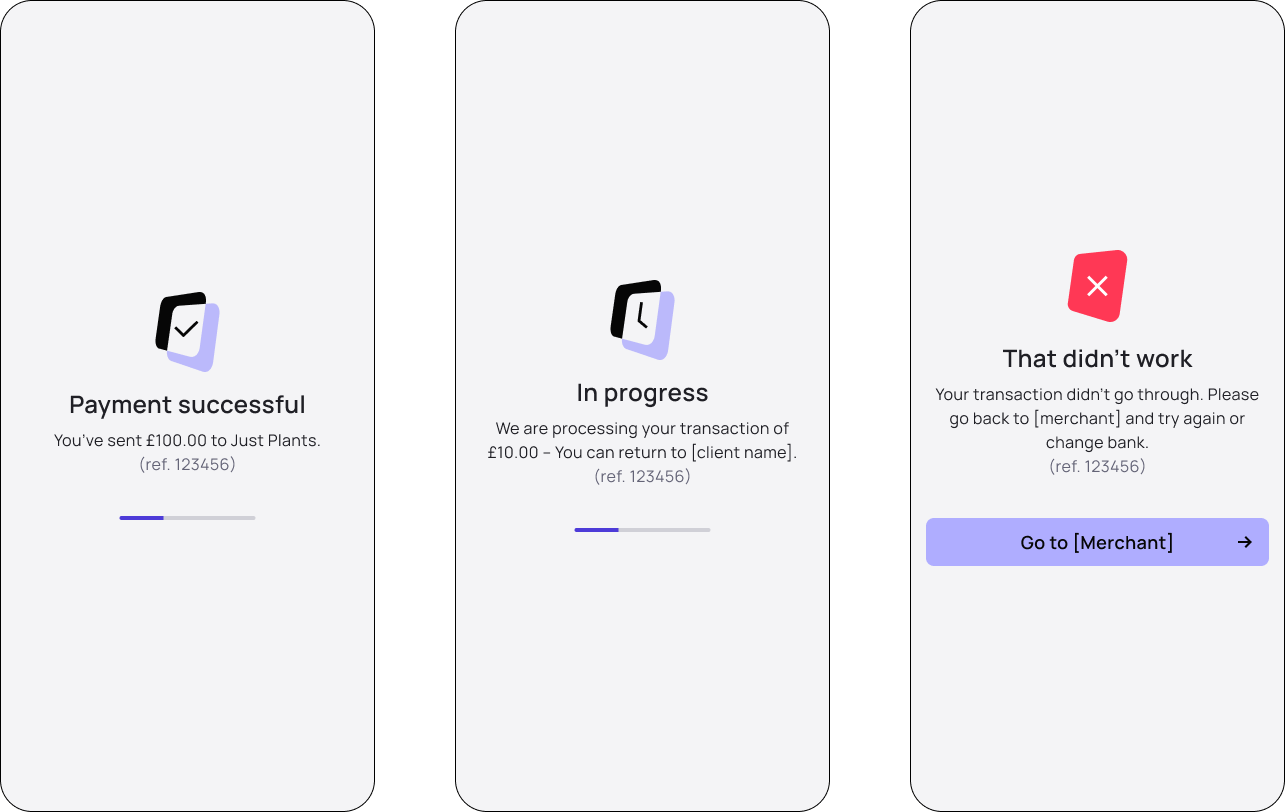
The payment result screen for successful, pending, and failed payments.
If you don't include this field, the default waiting period is 4 seconds. The maximum waiting period that you can set is 60 seconds.
This is an example of the maxWaitForResult
field within an integration, with a waiting period of up to 40 seconds.
} = initWebSdk({
maxWaitForResult: 40,
uiSettings: {
size: 'small',
recommendedPaymentMethod: true,
Switch between sandbox and production modes
By default, the Web SDK is set to sandbox mode.
To switch to production, include a production
field within the initWebSdk
object and set it to true
.
Below is an example of the production
mode turned on:
} = initWebSdk({
maxWaitForResult: 40,
production: true,
When a user makes a second payment on your checkout, the user flow changes. See our Web SDK demo to see the different experience for a first time user, or returning user with saved details:
Full request example
This is an example of the web SDK within an integration, with UI settings and callbacks configured.
import { initWebSdk } from '@truelayer-web-sdk'
const {
// Function to mount the container where the Pay button will be
mount,
// Function to initiate the Pay button with a payment
start,
// Function to remove event listeners used by the SDK, when the SDK is not longer needed.
cleanup,
// Function to dynamically open the learn more modal
openLearnMoreModal,
} = initWebSdk({
onDone: info => {
console.log(`Payment status: ${info.resultStatus}`)
},
onCancel: () => {
console.log('Payment cancelled')
},
onError: error => {
console.error('Payment error:', error)
},
onPayButtonClicked: () => {
console.log('Pay button clicked')
},
onNavigation: page => {
console.log('Page:', page)
},
onExpired: () => {
console.log('The payment has expired')
},
uiSettings: {
recommendedPaymentMethod: true,
size: 'large',
borderRadius: 8,
},
production: false,
})
Updated 21 days ago