Embedded payment page
Learn the basics of the embedded payment page.
New user? Go to the Web SDK docs
If you are integrating for the first time, and want to use an embedded payments integration, use the web and mobile SDKs instead.
The embedded payment page (EPP) is a prebuilt user interface that speeds up the integration process. Unlike the HPP the EPP runs entirely within your website as an overlay or inline on your page.
The EPP also features QR code authentication to enable desktop users to authorise payments with their mobile banking app. In addition to this, it has built-in localisation, covering local languages and authentication flows across Europe, helping you accept payments in more countries.
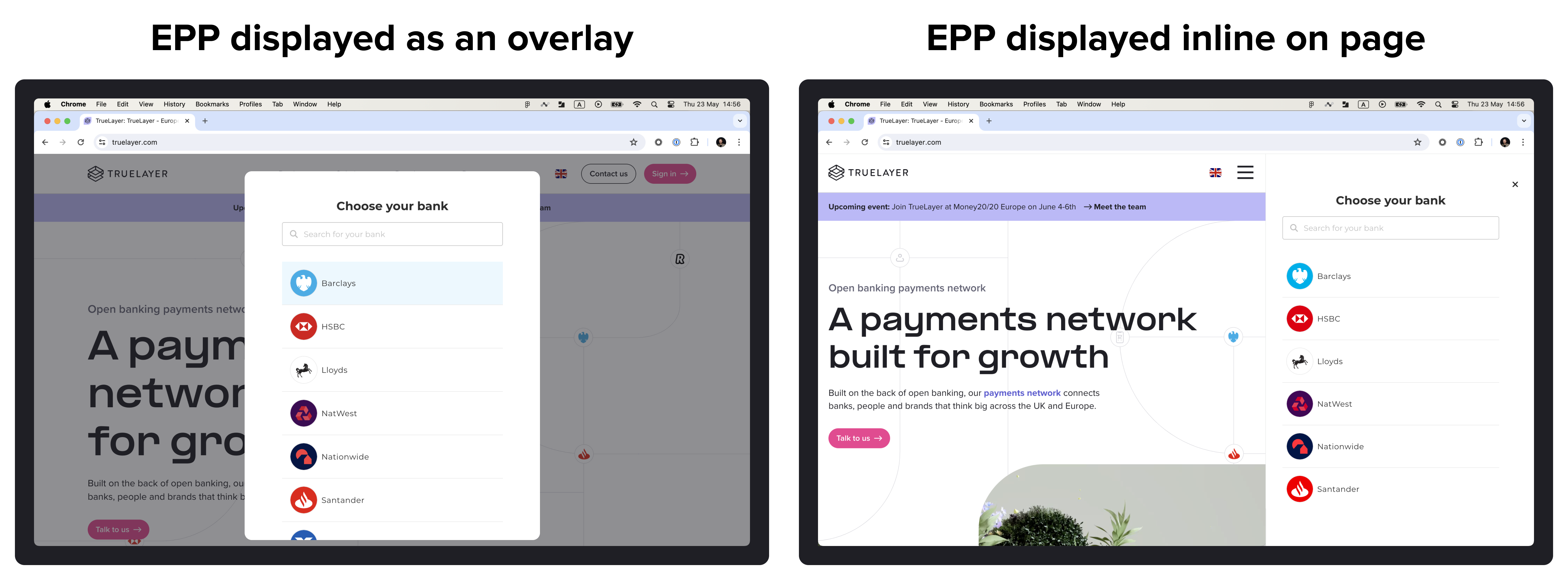
An example of the provider selection screen as an overlay (left) or inline (right) with the EPP.
EPP features
The EPP offers all of the functionality of the HPP, but embedded in your website or application. As such, the EPP offers:
- A bank selection screen.
- A scheme selection screen for EUR payments, if the bank supports multiple payment schemes.
- A consent screen.
- Collecting any additional inputs needed from your user, such as IBAN or branch code.
- A QR code that allows desktop users to continue the payment on their mobile phone.
- Functionality that enables users to authorise payments, such as:
- redirecting the user to their bank to authorise the payment.
- using embedded payment authorisation for banks that need it.
- Built-in payment and mandate result screens to inform of success, or to help users with failed payments or mandates.
A benefit of the EPP over a direct API integration is that if any new features are added, you can take advantage of them with minimal or no integration needed.
Supported countries
The EPP is currently optimised for the UK, Ireland, France, Germany, Spain, Finland, the Netherlands, and Lithuania.
Beta testers can also use the EPP for banks in Austria, Belgium, Poland and Portugal.
The user interface can be displayed in English, Spanish, French, Italian, German, Dutch, Portuguese, Polish, Finnish and Lithuanian.
Supported browsers
We've built the EPP to work best on:
- Chrome
- Edge
- Firefox
- Safari
You can also use the EPP in a mobile web view. If you display the EPP as an overlay, it will cover most of the screen, leaving a border so that your user can see that they're still on your site.
EPP payment journey
When a customer makes a payment through the embedded payment page, it follows this flow:
- The user selects the Pay by bank option or equivalent in your app or website.
- Your integration creates a payment with TrueLayer and gets a payment
id
and resource_token in response. - You initialise the EPP using this
id
andresource_token
and the EPP displays as an overlay or inline within your app or website. - Your user selects their bank on the screen.
- If a bank is unavailable it's greyed out on the provider selection screen, so the user can attempt to use a different bank.
- If your user is paying internationally from certain French or Finnish banks, a screen displays that explains how to enable international payments.
- Your user enters any additional information that the bank requires, and confirms.
If the user is making a EUR payment through a provider that supports multiple payment schemes, they select a scheme at this point. - If their bank supports app-to-app authentication, desktop users see a QR code. Scanning the code enables them to continue the payment using the banking app on their phone. They can also continue on desktop.
- The EPP redirects your user to their bank's website or app.
- Your user authorises the payment in their bank's website or app.
If the user needs to complete SCA as part of an embedded payment, they do so here. - Once the authorisation is complete, the bank redirects the user to the EPP's payment result screen.
- After the user acknowledges the payment result screen, they are redirected to the return URI that you set in Console.
As well as payments, your users can authorise mandates with the embedded payment page.
Use the EPP in your integration
Learn how to configure the authorisation flow for payments with the EPP.
Learn how to keep the EPP up to date, and use
Learn how to change the look and feel of the EPP to match your branding.
Learn how to use the EPP Demo page in Console to test, customise and set up your EPP.
Updated 23 days ago